こんにちは、ゆたんぽです。
本日は、Prismでもよく使用するListBox,ComboBoxのデータバインディングを説明していきます。
目次
ListBoxのデータバインディング
実際にListBoxを使用したデータバインディングを行います。
View4の作成
まずは、画面を作成します。
まずは、今まで通りPrismのUserControlを作成しましょう。
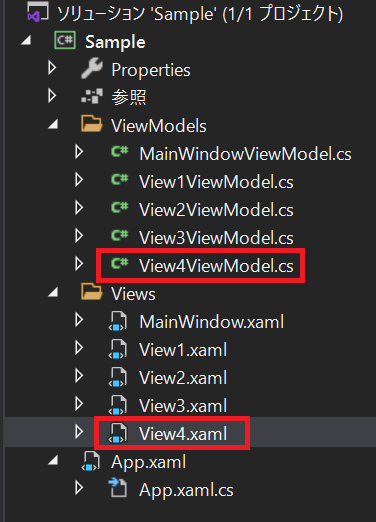
View4.xamlの実装
<UserControl x:Class="Sample.Views.View4"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True">
<Grid>
<ListBox ItemsSource="{Binding ListBox}"
Height="100"
Width="200"
Margin="10"/>
</Grid>
</UserControl>
上記のようにListBoxを作成します。
データバインディングは今までと違い、ItemResourceで行います。
Hieghtは高さで、Widthは幅を指定しています。
Marginは余白を設定しています。
View4ViewModel.csの実装
using Prism.Commands;
using Prism.Mvvm;
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
namespace Sample.ViewModels
{
public class View4ViewModel : BindableBase
{
public View4ViewModel()
{
_listBoxAdd.Add("じゃがいも");
_listBoxAdd.Add("にんじん");
_listBoxAdd.Add("にく");
_listBoxAdd.Add("ぱぷりか");
}
private ObservableCollection<string> _listBoxAdd = new ObservableCollection<string>();
public ObservableCollection<string> ListBoxAdd
{
get { return _listBoxAdd; }
set { SetProperty(ref _listBoxAdd, value); }
}
}
}
上記のようにViewで作成した,ListBoxのデータバインディングを行います。
ListBoxは、ObserableCollectionの型で指定します。
ObserableCollection は、項目が追加または削除されたとき、リスト全体が更新されたときに通知を行う動的なデータ コレクションを表します。
複数データを取り扱うものは、 ObserableCollection を使用するという感覚でよいと思います。
コンストラクタでは、ListBoxAddにデータを追加していきます。
データ追加は、List型と同じようにAddメソッドを使用します。
追加項目は、なんでも大丈夫です。
MainWindow.xamlの実装
<Window x:Class="Sample.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True"
Title="{Binding Title}" Height="350" Width="525"
WindowStartupLocation="CenterScreen">
<Grid>
<StackPanel>
<Label Content="システム日時"/>
<Label Content="{Binding SystemData}"/>
<Button Content="時間更新"
Command="{Binding UpdateButton}"/>
<Button Content="View1"
Command="{Binding ShowView1Button}"/>
<Button Content="View2"
Command="{Binding ShowView2Button}"/>
<Button Content="View3"
Command="{Binding ShowView3Button}"/>
<Button Content="View4"
Command="{Binding ShowView4Button}"/>
<ContentControl prism:RegionManager.RegionName="ContentRegion" />
</StackPanel>
</Grid>
</Window>
上記のようにView4を表示するためのボタンを追加してください。
何度も行ってきたので説明は割愛します。
MainWindowViewModel.csの実装
using Prism.Commands;
using Prism.Mvvm;
using Prism.Regions;
using Prism.Services.Dialogs;
using Sample.Views;
using System;
namespace Sample.ViewModels
{
public class MainWindowViewModel : BindableBase
{
private readonly IRegionManager _regionManager;
private readonly IDialogService _dialogService;
private string _title = "Sample Application";
/////////////////////////////////////省略////////////////////////////////////////////
public DelegateCommand ShowView4Button { get; }
public MainWindowViewModel(IRegionManager regionManager,IDialogService dialogService) //③
{
_regionManager = regionManager;
_dialogService = dialogService;
UpdateButton = new DelegateCommand(UpdateButtonExe);
ShowView1Button = new DelegateCommand(ShowView1ButtonExe);
ShowView2Button = new DelegateCommand(ShowView2ButtonExe);
ShowView3Button = new DelegateCommand(ShowView3ButtonExe);
ShowView4Button = new DelegateCommand(ShowView4ButtonExe);
}
/////////////////////////////////////省略////////////////////////////////////////////
private void ShowView4ButtonExe()
{
_regionManager.RequestNavigate("ContentRegion", nameof(View4));
}
}
}
先ほどMainWindow.xaml側で追加した「ShowView4Button」を実装していきます。
今まで通りなので、コピペを使ってパパっと終わらせてしまいましょう。
App.xaml.csの実装
using Sample.Views;
using Prism.Ioc;
using Prism.Modularity;
using System.Windows;
using Sample.ViewModels;
namespace Sample
{
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class App
{
protected override Window CreateShell()
{
return Container.Resolve<MainWindow>();
}
protected override void RegisterTypes(IContainerRegistry containerRegistry)
{
containerRegistry.RegisterForNavigation<View1>();
containerRegistry.RegisterForNavigation<View2>();
containerRegistry.RegisterForNavigation<View4>();
containerRegistry.RegisterDialog<View3, View3ViewModel>();
}
}
}
上記のようにApp.xaml.csに画面遷移するViewの名前を宣言します。
ここまで出来たら起動してみてください。
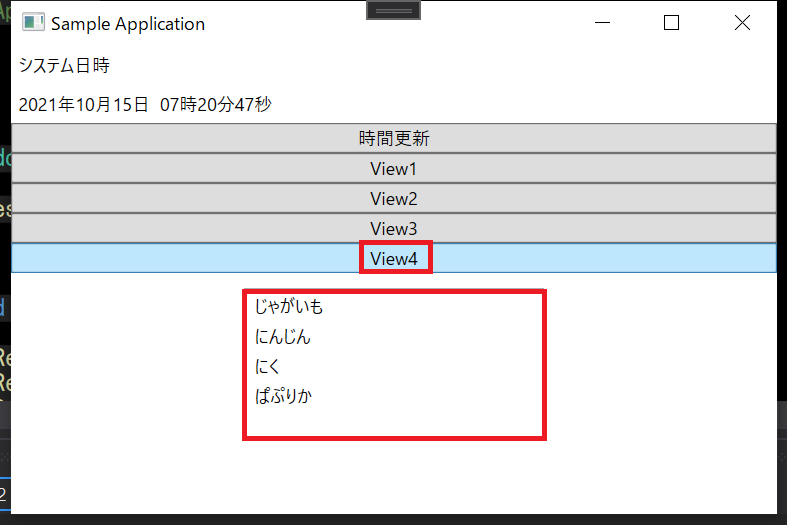
ComboBoxのデータバインディング
続いてComboBoxのデータバインディングを行います。ListBoxとほぼ同様の内容です。
View4.xamlの編集
<UserControl x:Class="Sample.Views.View4"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True">
<Grid>
<StackPanel>
<ListBox ItemsSource="{Binding ListBoxAdd}"
Height="100"
Width="200"
Margin="10"/>
<ComboBox ItemsSource="{Binding ConboBoxAdd}"/>
</StackPanel>
</Grid>
</UserControl>
ListBox同様、ItemSourceにデータバインディングしていきます。
MainWindow.xamlの実装
<Window x:Class="Sample.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True"
Title="{Binding Title}" Height="500" Width="525"
WindowStartupLocation="CenterScreen">
<Grid>
<StackPanel>
<Label Content="システム日時"/>
<Label Content="{Binding SystemData}"/>
<Button Content="時間更新"
Command="{Binding UpdateButton}"/>
<Button Content="View1"
Command="{Binding ShowView1Button}"/>
<Button Content="View2"
Command="{Binding ShowView2Button}"/>
<Button Content="View3"
Command="{Binding ShowView3Button}"/>
<Button Content="View4"
Command="{Binding ShowView4Button}"/>
<ContentControl prism:RegionManager.RegionName="ContentRegion" />
</StackPanel>
</Grid>
</Window>
ComboBoxを追加したことによって、縦の表示が見切れてしまうのでHeightを使用して、500へ高さを変更します。
View4ViewModel.csの実装
using Prism.Commands;
using Prism.Mvvm;
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
namespace Sample.ViewModels
{
public class View4ViewModel : BindableBase
{
public View4ViewModel()
{
_listBoxAdd.Add("じゃがいも");
_listBoxAdd.Add("にんじん");
_listBoxAdd.Add("にく");
_listBoxAdd.Add("ぱぷりか");
_comboBoxAdd.Add("オレンジ");
_comboBoxAdd.Add("グレープ");
_comboBoxAdd.Add("ピーチ");
_comboBoxAdd.Add("メロン");
}
private ObservableCollection<string> _listBoxAdd = new ObservableCollection<string>();
public ObservableCollection<string> ListBoxAdd
{
get { return _listBoxAdd; }
set { SetProperty(ref _listBoxAdd, value); }
}
private ObservableCollection<string> _comboBoxAdd = new ObservableCollection<string>();
public ObservableCollection<string> ComboBoxAdd
{
get { return _comboBoxAdd; }
set { SetProperty(ref _comboBoxAdd, value); }
}
}
}
ListBoxと同様にComboBoxAddという名前で、データバインディングしていきます。
コンストラクタでも任意の名前を追加していきましょう。
ここまで来たらデバッグを開始してみてください。
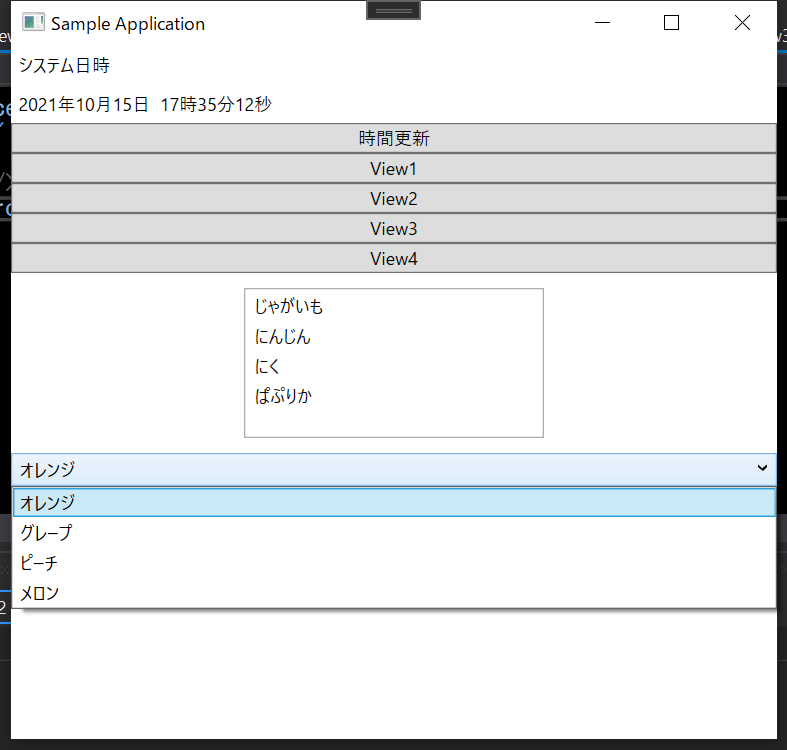
追加した名前がComboBoxで表示されていれば成功です。
まとめ&次回予告
今回は、ListBoxとComboBoxのデータバインディングを説明してきました。
どちらもItemSourceとObserableCollectionを使用することでデータバインドすることができました。
利用頻度が高いので覚えてみてください。
次回は、「ボタン押下可否のデータバインディング」を行っていきます。
ボタンを押すことをできなくしたりする内容です。
次回もよろしくお願いします。
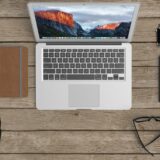